Importing UI from Figma
In this module, you will learn how to import the UI from Figma.
Figma is a collaborative app UI design tool that allows users to create, share, and comment on designs in real-time. It then allows exporting the UI in selected markup language for developers to carry on with the pre-designed app.
Uno Platform offers a Figma plugin that enables exporting the UI designed in Figma as both XAML and C# Markup. In this module, you'll learn how to export the UI from a pre-existing Figma design for the SimpleCalculator app, and how to import it into the app you've started to create in the previous modules.
Open the Figma file and set up an account
Open the SimpleCalculator Figma file via this link.
If you are not signed in with Figma, you will be asked to do so. Create an account if you don't have one. Follow the instructions to sign up with your Google account or use a username and password.
Install the Uno Platform plugin for Figma
The SimpleCalculator design will open in Figma, displaying the Cover page:
To export the UI from Figma, you'll need to install the Uno Platform plugin. To enable that you will need to create your own copy of the SimpleCalculator design.
In the top menu in the center of the screen click the little down arrow and select Duplicate to your drafts from the menu.The duplicated file will open in a new tab and additional menu items and controls will appear on the Figma ribbon.
In the search box enter "Uno Platform (Figma-To-XAML)". Launch the plugin by clicking the Uno Platform plugin.
Export the UI
- The page designed in the SimpleCalculator design in Figma is listed in the left-side navigation bar. Select Simple Calculator design, then select Simple Calc (either Dark or Light version) option that appears at the bottom of the sidebar.
- Open the Export tab, select C#, then click Refresh (the circled arrow button on the bottom).
- Select all contents starting from the line following
this
and ending at the semicolon (;).
MainPage.cs code contents (collapsed for brevity)
using Microsoft.UI.Xaml;
using Microsoft.UI.Xaml.Controls;
using Microsoft.UI.Xaml.Controls.Primitives;
using Microsoft.UI.Xaml.Media;
using System;
using Uno.Extensions.Markup;
using Uno.Material;
using Uno.Themes;
using Uno.Toolkit.UI;
namespace SimpleCalculator;
public partial class MainPage : Page
{
public MainPage()
{
this
.Background(Theme.Brushes.Background.Default)
.StatusBar(foreground: StatusBarForegroundTheme.Light)
.Resources
(
r => r
.Add("Icon_MoonIcon","F1 M 10.34000015258789 0.006284978240728378 C 4.590000152587891 -0.19371502473950386 0 4.406285285949707 0 9.986285209655762 C 0 15.506285190582275 4.480000019073486 19.986284255981445 10 19.986284255981445 C 13.710000038146973 19.986284255981445 16.929999828338623 17.96628475189209 18.65999984741211 14.96628475189209 C 11.149999618530273 14.71628475189209 6.570000171661377 6.536285188049078 10.34000015258789 0.006284978240728378 Z")
.Add("Icon_SunIcon","F1 M 5.760000228881836 4.289999961853027 L 3.9600000381469727 2.5 L 2.549999952316284 3.9100000858306885 L 4.340000152587891 5.699999809265137 L 5.760000228881836 4.289999961853027 Z M 3 9.949999809265137 L 0 9.949999809265137 L 0 11.949999809265137 L 3 11.949999809265137 L 3 9.949999809265137 Z M 12 0 L 10 0 L 10 2.950000047683716 L 12 2.950000047683716 L 12 0 L 12 0 Z M 19.450000762939453 3.9100000858306885 L 18.040000915527344 2.5 L 16.25 4.289999961853027 L 17.65999984741211 5.699999809265137 L 19.450000762939453 3.9100000858306885 Z M 16.239999771118164 17.610000610351562 L 18.030000686645508 19.40999984741211 L 19.440000534057617 18 L 17.639999389648438 16.21000099182129 L 16.239999771118164 17.610000610351562 Z M 19 9.949999809265137 L 19 11.949999809265137 L 22 11.949999809265137 L 22 9.949999809265137 L 19 9.949999809265137 Z M 11 4.949999809265137 C 7.690000057220459 4.949999809265137 5 7.639999866485596 5 10.949999809265137 C 5 14.259999752044678 7.690000057220459 16.950000762939453 11 16.950000762939453 C 14.309999942779541 16.950000762939453 17 14.259999752044678 17 10.949999809265137 C 17 7.639999866485596 14.309999942779541 4.949999809265137 11 4.949999809265137 Z M 10 21.900001525878906 L 12 21.900001525878906 L 12 18.950000762939453 L 10 18.950000762939453 L 10 21.900001525878906 Z M 2.549999952316284 17.990001678466797 L 3.9600000381469727 19.400001525878906 L 5.75 17.600000381469727 L 4.340000152587891 16.190000534057617 L 2.549999952316284 17.990001678466797 Z")
)
.Content
(
new AutoLayout()
.Background(Theme.Brushes.Secondary.Container.Default)
.Children
(
new AutoLayout()
.MaxWidth(700)
.AutoLayout(primaryAlignment: AutoLayoutPrimaryAlignment.Stretch)
.Children
(
new ToggleButton()
.Background(Theme.Brushes.Surface.Default)
.Margin(10,24)
.CornerRadius(20)
.AutoLayout(counterAlignment: AutoLayoutAlignment.Center)
.Content
(
new PathIcon()
.Data(StaticResource.Get<Geometry>("Icon_SunIcon"))
.Foreground(Theme.Brushes.Primary.Default)
)
.ControlExtensions
(
alternateContent:
new PathIcon()
.Data(StaticResource.Get<Geometry>("Icon_MoonIcon"))
.Foreground(Theme.Brushes.Primary.Default)
),
new AutoLayout()
.Spacing(16)
.PrimaryAxisAlignment(AutoLayoutAlignment.End)
.Padding(16,8)
.MinHeight(120)
.AutoLayout(primaryAlignment: AutoLayoutPrimaryAlignment.Stretch)
.Children
(
new TextBlock()
.Text(b => b.Binding("Calculator.Equation"))
.Foreground(Theme.Brushes.OnSecondary.Container.Default)
.Style(Theme.TextBlock.Styles.DisplaySmall)
.AutoLayout(counterAlignment: AutoLayoutAlignment.End),
new TextBlock()
.Text(b => b.Binding("Calculator.Output"))
.Foreground(Theme.Brushes.OnBackground.Default)
.Style(Theme.TextBlock.Styles.DisplayLarge)
.AutoLayout(counterAlignment: AutoLayoutAlignment.End)
),
new AutoLayout()
.Spacing(16)
.PrimaryAxisAlignment(AutoLayoutAlignment.End)
.Padding(16)
.Children
(
new AutoLayout()
.Spacing(16)
.Orientation(Orientation.Horizontal)
.Height(72)
.Children
(
new Button()
.Background(Theme.Brushes.OnSurface.Inverse.Default)
.Content("C")
.Height(72)
.CornerRadius(24)
.Style(Theme.Button.Styles.FilledTonal)
.AutoLayout
(
counterAlignment: AutoLayoutAlignment.Start,
primaryAlignment: AutoLayoutPrimaryAlignment.Stretch
),
new Button()
.Background(Theme.Brushes.OnSurface.Inverse.Default)
.Content("+/-")
.Height(72)
.CornerRadius(24)
.Style(Theme.Button.Styles.FilledTonal)
.AutoLayout
(
counterAlignment: AutoLayoutAlignment.Start,
primaryAlignment: AutoLayoutPrimaryAlignment.Stretch
),
new Button()
.Background(Theme.Brushes.OnSurface.Inverse.Default)
.Content("%")
.Height(72)
.CornerRadius(24)
.Style(Theme.Button.Styles.FilledTonal)
.AutoLayout
(
counterAlignment: AutoLayoutAlignment.Start,
primaryAlignment: AutoLayoutPrimaryAlignment.Stretch
),
new Button()
.Content("÷")
.Height(72)
.CornerRadius(24)
.AutoLayout
(
counterAlignment: AutoLayoutAlignment.Start,
primaryAlignment: AutoLayoutPrimaryAlignment.Stretch
)
),
new AutoLayout()
.Spacing(16)
.Orientation(Orientation.Horizontal)
.Height(72)
.Children
(
new Button()
.Background(Theme.Brushes.Surface.Default)
.Content("7")
.Height(72)
.MinWidth(72)
.CornerRadius(24)
.Style(Theme.Button.Styles.FilledTonal)
.AutoLayout
(
counterAlignment: AutoLayoutAlignment.Start,
primaryAlignment: AutoLayoutPrimaryAlignment.Stretch
),
new Button()
.Background(Theme.Brushes.Surface.Default)
.Content("8")
.Height(72)
.MinWidth(72)
.CornerRadius(24)
.Style(Theme.Button.Styles.FilledTonal)
.AutoLayout
(
counterAlignment: AutoLayoutAlignment.Start,
primaryAlignment: AutoLayoutPrimaryAlignment.Stretch
),
new Button()
.Background(Theme.Brushes.Surface.Default)
.Content("9")
.Height(72)
.MinWidth(72)
.CornerRadius(24)
.Style(Theme.Button.Styles.FilledTonal)
.AutoLayout
(
counterAlignment: AutoLayoutAlignment.Start,
primaryAlignment: AutoLayoutPrimaryAlignment.Stretch
),
new Button()
.Content("×")
.Height(72)
.MinWidth(72)
.CornerRadius(24)
.AutoLayout
(
counterAlignment: AutoLayoutAlignment.Start,
primaryAlignment: AutoLayoutPrimaryAlignment.Stretch
)
),
new AutoLayout()
.Spacing(16)
.Orientation(Orientation.Horizontal)
.Height(72)
.Children
(
new Button()
.Background(Theme.Brushes.Surface.Default)
.Content("4")
.Height(72)
.MinWidth(72)
.CornerRadius(24)
.Style(Theme.Button.Styles.FilledTonal)
.AutoLayout
(
counterAlignment: AutoLayoutAlignment.Start,
primaryAlignment: AutoLayoutPrimaryAlignment.Stretch
),
new Button()
.Background(Theme.Brushes.Surface.Default)
.Content("5")
.Height(72)
.MinWidth(72)
.CornerRadius(24)
.Style(Theme.Button.Styles.FilledTonal)
.AutoLayout
(
counterAlignment: AutoLayoutAlignment.Start,
primaryAlignment: AutoLayoutPrimaryAlignment.Stretch
),
new Button()
.Background(Theme.Brushes.Surface.Default)
.Content("6")
.Height(72)
.MinWidth(72)
.CornerRadius(24)
.Style(Theme.Button.Styles.FilledTonal)
.AutoLayout
(
counterAlignment: AutoLayoutAlignment.Start,
primaryAlignment: AutoLayoutPrimaryAlignment.Stretch
),
new Button()
.Content("−")
.Height(72)
.MinWidth(72)
.CornerRadius(24)
.AutoLayout
(
counterAlignment: AutoLayoutAlignment.Start,
primaryAlignment: AutoLayoutPrimaryAlignment.Stretch
)
),
new AutoLayout()
.Spacing(16)
.Orientation(Orientation.Horizontal)
.Height(72)
.Children
(
new Button()
.Background(Theme.Brushes.Surface.Default)
.Content("1")
.Height(72)
.MinWidth(72)
.CornerRadius(24)
.Style(Theme.Button.Styles.FilledTonal)
.AutoLayout
(
counterAlignment: AutoLayoutAlignment.Start,
primaryAlignment: AutoLayoutPrimaryAlignment.Stretch
),
new Button()
.Background(Theme.Brushes.Surface.Default)
.Content("2")
.Height(72)
.MinWidth(72)
.CornerRadius(24)
.Style(Theme.Button.Styles.FilledTonal)
.AutoLayout
(
counterAlignment: AutoLayoutAlignment.Start,
primaryAlignment: AutoLayoutPrimaryAlignment.Stretch
),
new Button()
.Background(Theme.Brushes.Surface.Default)
.Content("3")
.Height(72)
.MinWidth(72)
.CornerRadius(24)
.Style(Theme.Button.Styles.FilledTonal)
.AutoLayout
(
counterAlignment: AutoLayoutAlignment.Start,
primaryAlignment: AutoLayoutPrimaryAlignment.Stretch
),
new Button()
.Content("+")
.Height(72)
.MinWidth(72)
.CornerRadius(24)
.AutoLayout
(
counterAlignment: AutoLayoutAlignment.Start,
primaryAlignment: AutoLayoutPrimaryAlignment.Stretch
)
),
new AutoLayout()
.Spacing(16)
.Orientation(Orientation.Horizontal)
.Height(72)
.Children
(
new Button()
.Background(Theme.Brushes.Surface.Default)
.Content(".")
.Height(72)
.MinWidth(72)
.CornerRadius(24)
.Style(Theme.Button.Styles.FilledTonal)
.AutoLayout
(
counterAlignment: AutoLayoutAlignment.Start,
primaryAlignment: AutoLayoutPrimaryAlignment.Stretch
),
new Button()
.Background(Theme.Brushes.Surface.Default)
.Content("0")
.Height(72)
.MinWidth(72)
.CornerRadius(24)
.Style(Theme.Button.Styles.FilledTonal)
.AutoLayout
(
counterAlignment: AutoLayoutAlignment.Start,
primaryAlignment: AutoLayoutPrimaryAlignment.Stretch
),
new Button()
.Background(Theme.Brushes.Surface.Default)
.Content("⌫")
.Height(72)
.MinWidth(72)
.CornerRadius(24)
.Style(Theme.Button.Styles.FilledTonal)
.AutoLayout
(
counterAlignment: AutoLayoutAlignment.Start,
primaryAlignment: AutoLayoutPrimaryAlignment.Stretch
),
new Button()
.Content("=")
.Height(72)
.MinWidth(72)
.CornerRadius(24)
.AutoLayout
(
counterAlignment: AutoLayoutAlignment.Start,
primaryAlignment: AutoLayoutPrimaryAlignment.Stretch
)
)
)
)
)
)
;
}
}
Copy the selected code to the clipboard (Ctrl+C on Windows).
Open MainPage.cs and replace all the Page contents with the copied code.
To set the appropriate font size for all buttons, access the MaterialFontsOverride.cs file in the Style folder. Go to the Figma Plugin, in the Export tab, and select Fonts Override File from the dropdown menu. Copy the content in the ResourceDictionary and replace it in your MaterialFontsOverride.cs.
MaterialFontsOverride.cs code contents (collapsed for brevity)
using Microsoft.UI.Xaml;
using Uno.Extensions.Markup;
namespace SimpleCalculator.Styles;
public partial class MaterialFontsOverride : ResourceDictionary
{
public MaterialFontsOverride()
{
this
.Build
(
r => r
.Add("LabelLargeFontSize",32)
)
;
}
}
- Now we need to prepare our UI with the Binding expressions that we will need in the App Architecture module. First let's add the
DataContext
to the page. To do so add.DataContext(new TempDataContext(), (page, vm) => page
before the.Content
definition. Ensure to properly terminate the DataContext with a closing)
preceding the semicolon at the end of the page's code.
this
.Background(Theme.Brushes.Background.Default)
.StatusBar(foreground: StatusBarForegroundTheme.Light)
.Resources
(
r => r
...
)
.DataContext(new TempDataContext(), (page, vm) => page
.Content
(
...
)
);
For all buttons we need to add Command(() => vm.InputCommand)
and CommandParameter
that will receive the same content as the Content
attribute. For example, the first button would then be:
new Button()
.Background(Theme.Brushes.OnSurface.Inverse.Default)
.Content("C")
.Height(72)
.CornerRadius(24)
.Style(Theme.Button.Styles.FilledTonal)
.AutoLayout
(
counterAlignment: AutoLayoutAlignment.Start,
primaryAlignment: AutoLayoutPrimaryAlignment.Stretch
)
.CommandParameter("C")
.Command(() => vm.InputCommand),
Apply these instructions to all the buttons on our page, except for the delete (back) button, which should display "⌫". Remember that for this specific button, set the CommandParameter
as the text "back".
Last we need to update our ToggleButton
with the Binding expression IsChecked(x => x.Binding(() => vm.IsDark).TwoWay())"
for the theme switching (Light and Dark).
new ToggleButton()
.Background(Theme.Brushes.Surface.Default)
.Margin(10,24)
.CornerRadius(20)
.AutoLayout(counterAlignment: AutoLayoutAlignment.Center)
.IsChecked(x => x.Binding(() => vm.IsDark).TwoWay())
.Content
(
...
)
.ControlExtensions
(
...
),
Run the app
Run the app (F5 on Visual Studio) and observe the UI, it should look similar to the following:
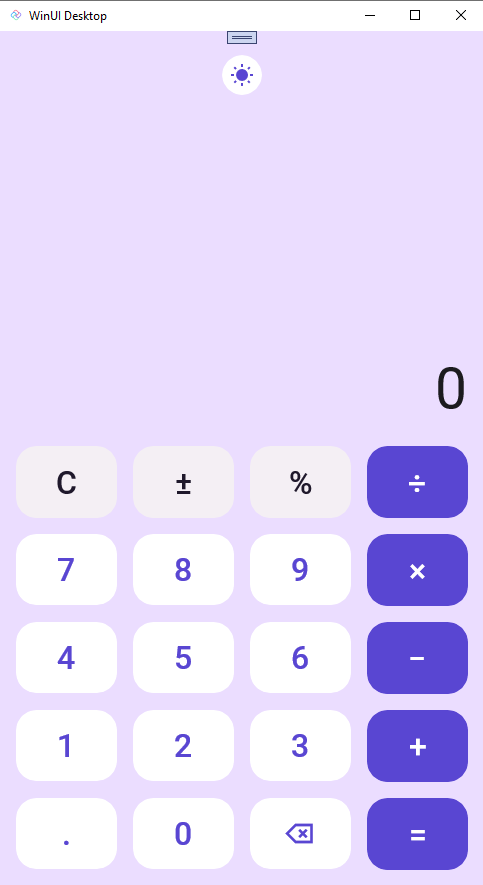
Next Step
The next module offers an alternative way to import the UI code without Figma. Since you've already imported the UI, you may skip to Module 4, where you will add the app architecture.